NumPy Arrays
Last Update Unknown
How can we use NumPy?
Array: An array is similar to a list but it's usually fixed in size and has all elements of the same type.
To use arrays in Python, we first need to import the NumPy module, which comes with all sorts of methods to manipulate arrays.
We can then create an array by:
Each element is of the same type, in this case integers:
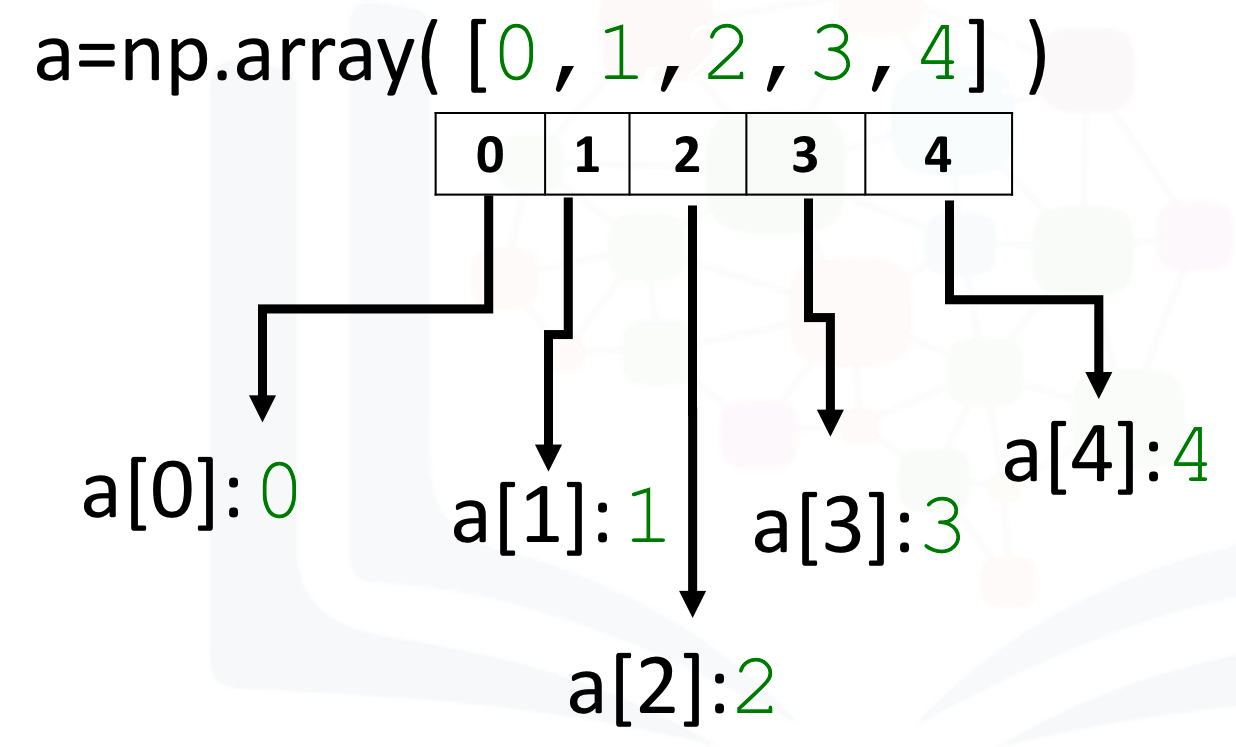
As with lists, we can access each element via a square bracket:
Type
If we check the type of the array we get numpy.ndarray:
As numpy arrays contain data of the same type, we can use the attribute "dtype" to obtain the Data-type of the array’s elements. In this case a 64-bit integer:
We can create a numpy array with real numbers:
When we check the type of the array we get numpy.ndarray:
If we examine the attribute dtype we see float 64, as the elements are not integers:
Assign Value
We can change the value of the array, consider the array c:
We can change the first element of the array to 100 as follows:
We can change the 5th element of the array to 0 as follows:
Slicing
Like in lists, we can slice NumPy arrays. For instance, we can select the elements from 1 to 3 and assign it to a new numpy array d as follows:
We can assign the corresponding indexes to new values as follows:
Assign Value with List
Let's use lists to select specific indexed elements. The list 'select' contains several values:
We can use the list as an argument in the brackets. The output is the elements corresponding to the particular index:
We can assign the specified elements to a new value. For example, we can assign the values to 100 000 as follows:
Other Attributes
Let's review some basic array attributes using the array a:
The attribute size is the number of elements in the array:
The next two attributes will make more sense when we get to higher dimensions but let's review them. The attribute ndim represents the number of array dimensions or the rank of the array, in this case, one:
The attribute shape is a tuple of integers indicating the size of the array in each dimension:
Simple operations on array of numbers
NumPy Array Operations
Array Addition
Consider the numpy array u:
Consider the numpy array v:
We can add the two arrays and assign it to z:
The operation is equivalent to vector addition
Array Multiplication
Consider the vector numpy array y:
We can multiply every element in the array by 2. In vectors/matrices terminoloty, **2** is a scalar.
This is equivalent to multiplying a vector by a scalar
Product of Two NumPy Arrays
Consider the following array u:
Consider the following array v:
The product of the two numpy arrays u and v is given by:
Dot Product
For 1D arrays (which is what we are dealing with right now) the dot product is the inner product of vectors.
The dot product of the two arrays u and v is given by:
u[0]*v[0] + u[1]*v[1] = 1x3 + 2x2 = 7
So the dot product of two 1D arrays creates a scalar.
Another example:
u = [2, 3, 4]
v = [5, 6, 7]
u[0]*v[0] + u[1]*v[1] + u[2]*v[2] = 2x5 + 3x6 + 4x7 = 10 + 18 + 28 = 56
Adding a constant to a NumPy array
Consider the following array:
Adding the constant 1 to each element in the array:
The process is summarised in the following animation:
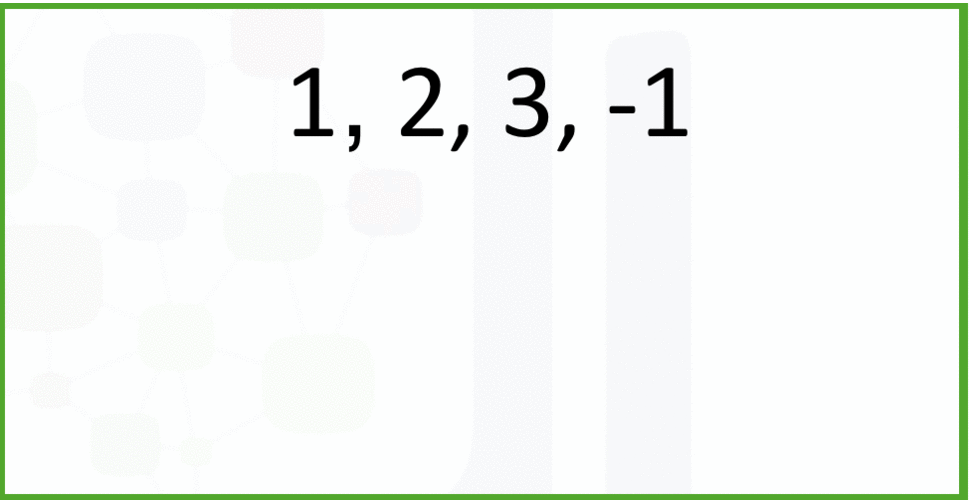
Mathematical Functions and Constants
We can access the value of pi in NumPy as follows :
We can create the following NumPy array in Radians:
We can apply the function sin to the array x and assign the values to the array y; this applies the sine function to each element in the array:
Linspace
A useful function for plotting mathematical functions is `linspace`. It returns evenly spaced numbers over a specified interval. We specify the starting point of the sequence and the ending point of the sequence. The parameter "num" indicates the Number of samples to generate, in this case 5:
If we change the parameter num to 9, we get 9 evenly spaced numbers over the interval from -2 to 2:
We can use the function line space to generate 100 evenly spaced samples from the interval 0 to 2π:
We can apply the sine function to each element in the array x and assign it to the array y: